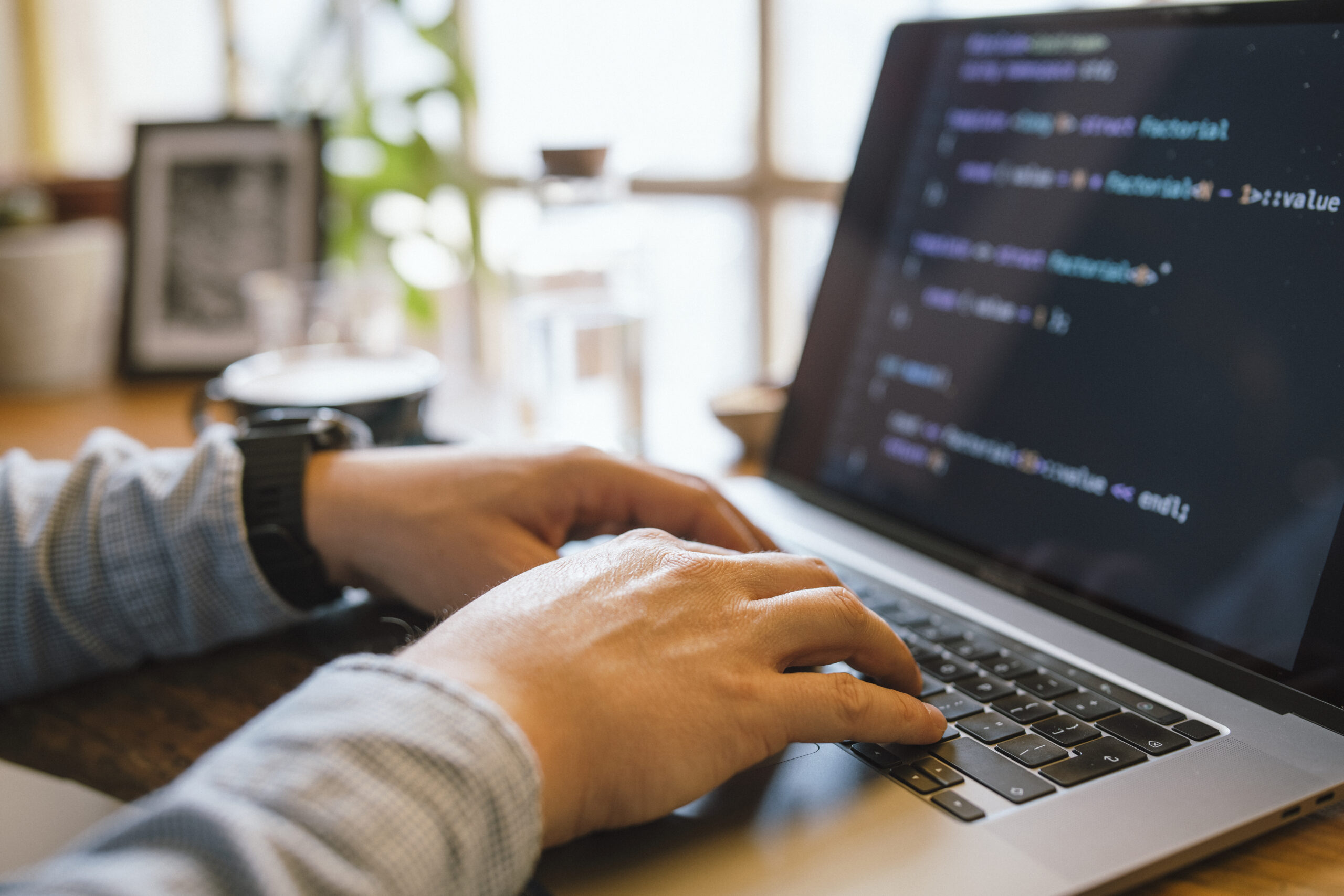
Debugging is One of the more essential — nevertheless typically missed — abilities within a developer’s toolkit. It's actually not pretty much correcting broken code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to unravel complications competently. Whether you're a beginner or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically increase your productiveness. Listed below are numerous techniques to assist developers stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of advancement, being aware of how you can interact with it proficiently for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few builders only scratch the floor of what these tools can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code about the fly. When utilised properly, they Permit you to observe particularly how your code behaves for the duration of execution, which is priceless for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-close builders. They allow you to inspect the DOM, monitor community requests, see authentic-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn frustrating UI challenges into manageable jobs.
For backend or system-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control in excess of running processes and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging efficiency challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Manage programs like Git to understand code background, uncover the precise minute bugs were being introduced, and isolate problematic variations.
Finally, mastering your applications means going past default settings and shortcuts — it’s about producing an personal expertise in your development atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote fixing the actual issue instead of fumbling via the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the situation. Ahead of jumping into the code or earning guesses, builders want to create a consistent ecosystem or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing a difficulty is gathering just as much context as you possibly can. Talk to issues like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the a lot easier it gets to isolate the precise circumstances less than which the bug occurs.
As you’ve collected more than enough details, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar knowledge, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the condition but additionally avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd come about only on certain operating techniques, browsers, or underneath particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a state of mind. It needs persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse far more Plainly using your crew or people. It turns an abstract criticism right into a concrete obstacle — Which’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you what exactly occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them initially.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Understanding to recognize these can substantially increase your debugging procedure.
Some problems are imprecise or generic, As well as in These situations, it’s crucial to examine the context through which the mistake happened. Check connected log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it provides actual-time insights into how an application behaves, aiding you realize what’s taking place under the hood with no need to pause execution or stage with the code line by line.
An excellent logging technique starts with understanding what to log and at what level. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for basic occasions (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure significant messages and decelerate your method. Focus on key situations, condition changes, enter/output values, and demanding conclusion factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
Eventually, intelligent logging is about balance and clarity. By using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a complex task—it's a type of investigation. To properly establish and fix bugs, developers should technique the method similar to a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness difficulties. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece jointly a transparent photo of what’s occurring.
Following, sort hypotheses. Question by yourself: What could possibly be leading to this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before below equivalent situations? The goal should be to slim down prospects and determine potential culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed setting. In the event you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, Gustavo Woltmann coding an off-by-one mistake, or a race issue. Be thorough and client, resisting the urge to patch the issue without having fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface afterwards.
And lastly, maintain notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and aid Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed difficulties in complex techniques.
Publish Checks
Writing tests is one of the best strategies to transform your debugging skills and General advancement effectiveness. Checks don't just help catch bugs early but will also function a security Web that offers you confidence when creating modifications in your codebase. A effectively-examined application is simpler to debug since it permits you to pinpoint just the place and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a test fails, you immediately know where to glimpse, appreciably cutting down enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously being preset.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other effortlessly. They’re specially helpful for catching bugs that manifest in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a element effectively, you would like to grasp its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when the issue is solved. This solution ensures that the identical bug doesn’t return Down the road.
In short, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to become immersed in the condition—staring at your display for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you simply wrote just hours before. During this point out, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also enable avoid burnout, Particularly during extended debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline is to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it surely really brings about faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart method. It presents your brain Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of solving it.
Understand From Each Bug
Each and every bug you face is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make much better coding behaviors transferring ahead.
Documenting bugs can be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've uncovered from a bug with your friends might be Specifically powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the similar concern boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your progress journey. In any case, some of the ideal builders will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur absent a smarter, a lot more able developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.